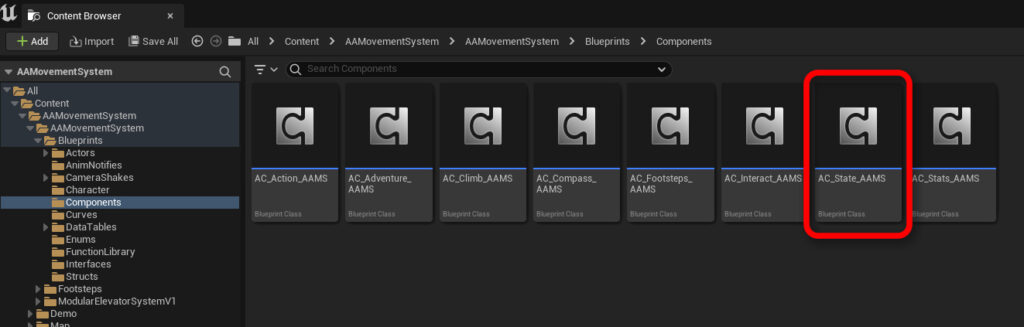
Introduction to the State Component #
The main component of AAMS (Action-Adventure Movement System) is the State Component. While utilizing all actor components is recommended to maximize AAMS’s potential, the State Component is the only one that is absolutely required. This component acts as the intermediary for the entire system, orchestrating the functionality of all other actor components. It manages the setting and getting of the Current Movement State and Movement Speed Mode, processes movement input, and configures the camera settings. This section provides a comprehensive overview of the State Component and its essential functions.
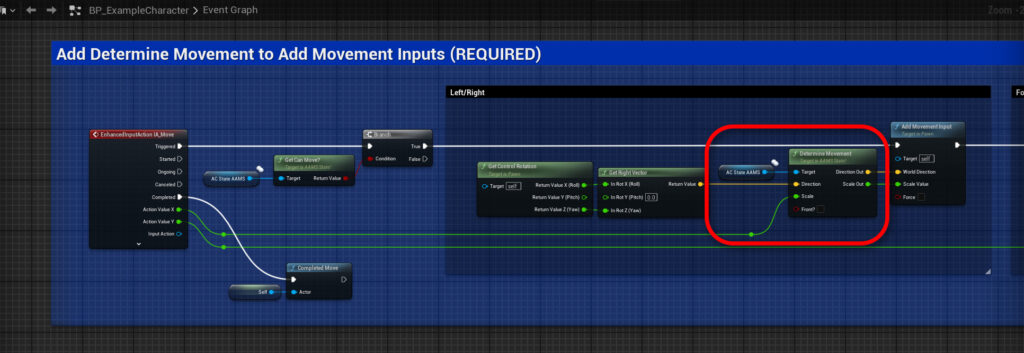
Determine Movement Input #
Instead of directly replacing the Add Movement Input in your character blueprint, AAMS employs a specialized function to determine the movement input direction and scale based on the current Movement State. The Determine Movement function takes your normal world direction and Action Value, used in the regular character blueprint, and communicates with the appropriate actor component to calculate the direction and scale to add into the Add Movement Input.
In the image below, you can see the Determine Movement function in action. The incoming movements use a switch based on the current Movement State and check the appropriate actor component before returning the value to the Add Movement Input in the character blueprint. If the current Movement State is equal to None, meaning there is no special AAMS movement occurring, the function will return the same values used in the character blueprint, without communicating with any other actor components.
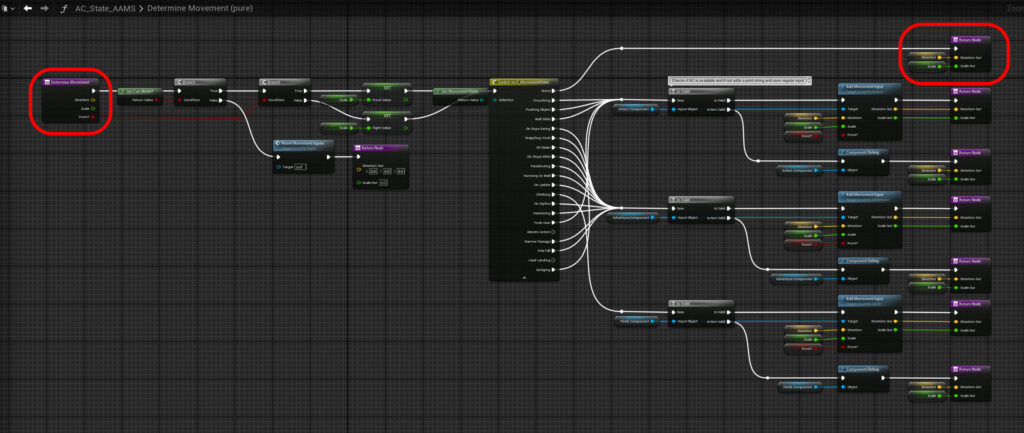
If the current Movement State is set to something other than None, the Determine Movement function ensures the appropriate actor component is added to the character blueprint. If the required actor component is not present, it returns the same values as received from the character blueprint. If the required actor component is present, the Determine Movement function begins communicating with the actor component’s Add Movement Input function to determine the needed output values.
Example: Crouching #
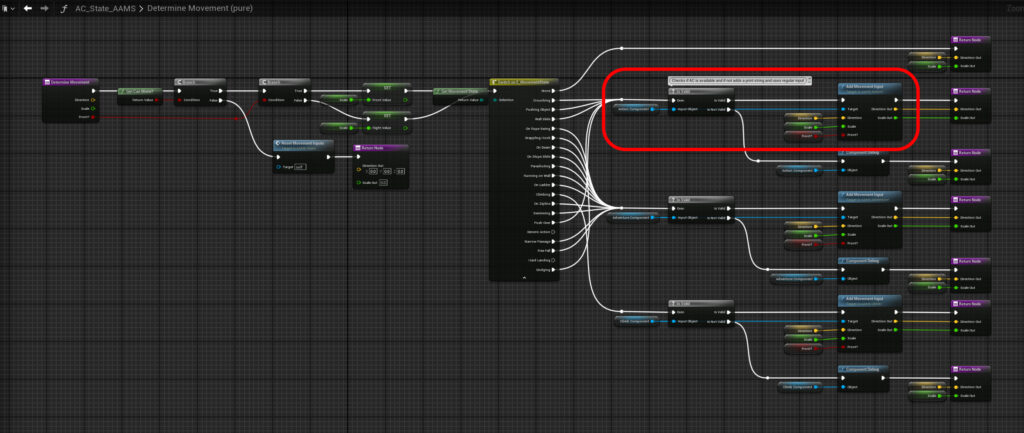
If the player is crouching, the Determine Movement function checks if the Action Component is present. If valid, it sends the incoming values to the Add Movement Input function inside the Action Component. The Add Movement Input function inside the Action Component is laid out similarly to the Determine Movement function. There is a switch for the current Movement State and functions to determine the necessary outgoing values. Currently, the crouching state does not alter incoming values, so the same values are used and sent to the Add Movement Input in the character blueprint.
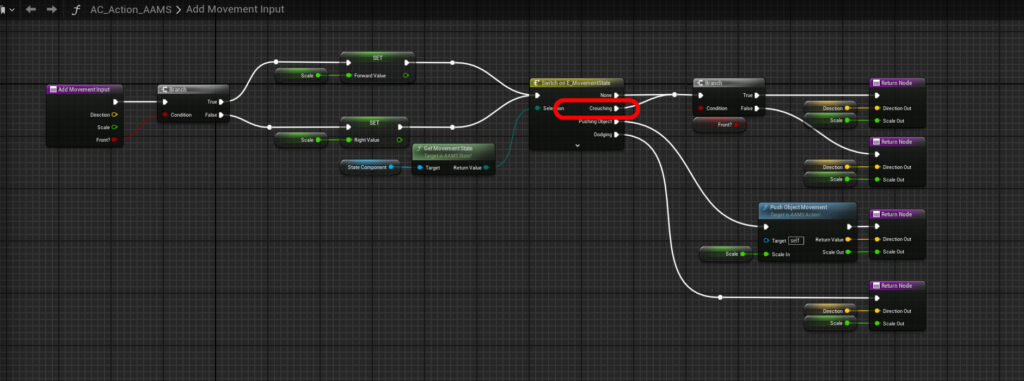
Example: Push Object #
For the Push Object state, before returning the values back to the State Component and then to the character blueprint, the values are sent to a function called Push Object Movement. This function runs blocking obstacle traces, determines movement poses, and calculates character speeds and locations before returning the new values to the Add Movement Input inside the character blueprint.
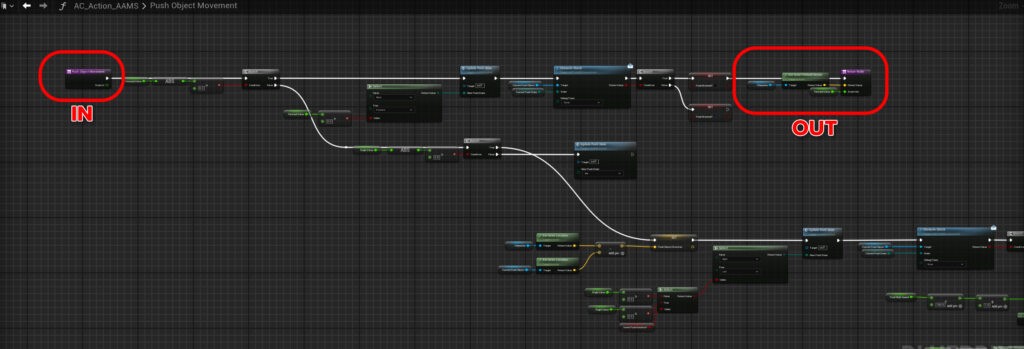
Movement State #
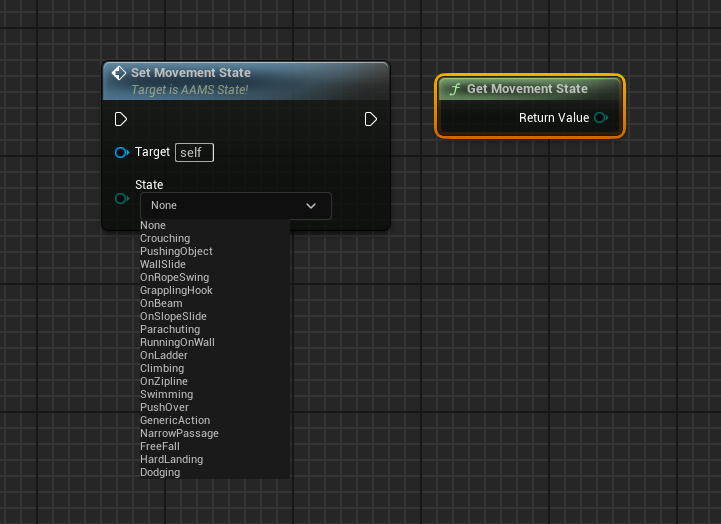
The Movement State is the foundation of the entire AAMS. The Movement State enum in the State Component is used by every element in the movement system, from the animation blueprint to the movement components, interaction system, and everything in between.
Set Movement State #
The Set Movement State function in the State Component is utilized by the Action Component, Adventure Component, and Climb Component when a new movement value is set. When the Set Movement State function is called, it ensures the incoming value differs from the current state. If true, it sends the new state to the character’s animation blueprint, triggers the On Movement State Changed Event dispatcher to update all accompanying blueprints (actor components, widgets, etc.), and lastly updates the camera structure, which will be discussed in a different section.

Get Movement State #
The Get Movement State function is a pure function used to retrieve the current value of the Movement State Enum. It can be called in any blueprint that has a State Component reference.
Move Speed Mode #
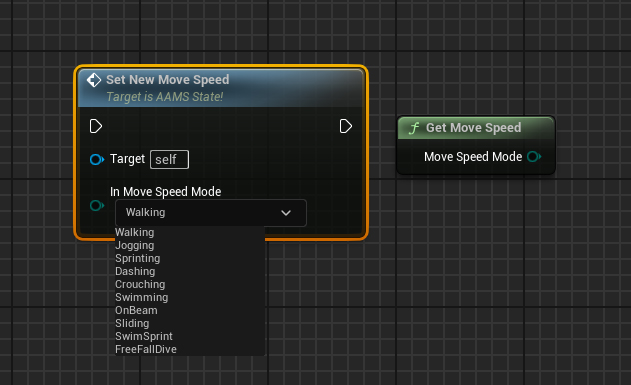
Move Speed Mode allows for multiple max speeds to be easily managed within one function, independent of Movement States. The Set New Move Speed function can be called to change the Max Walk Speed, Swim Speed, and Crouch Speed to the desired preset speeds.
Set Movement Speeds #
To set the desired move speed for a character, select the State Component in the character blueprint and open the Details tab. Scroll down to the Movement Speeds dropdown to configure the speeds.
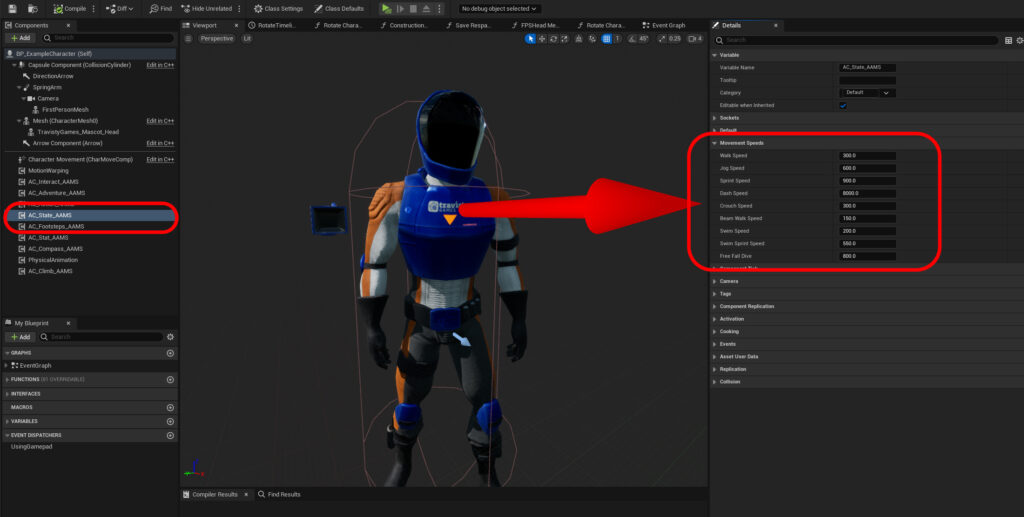
The current Movement Speed Modes include:
- Walk Speed
- Jog Speed
- Sprint Speed
- Dash Speed
- Crouch Speed
- Beam Walk Speed
- Swim Speed
- Swim Sprint Speed
- Free Fall Dive Speed
Camera Perspective & Settings #
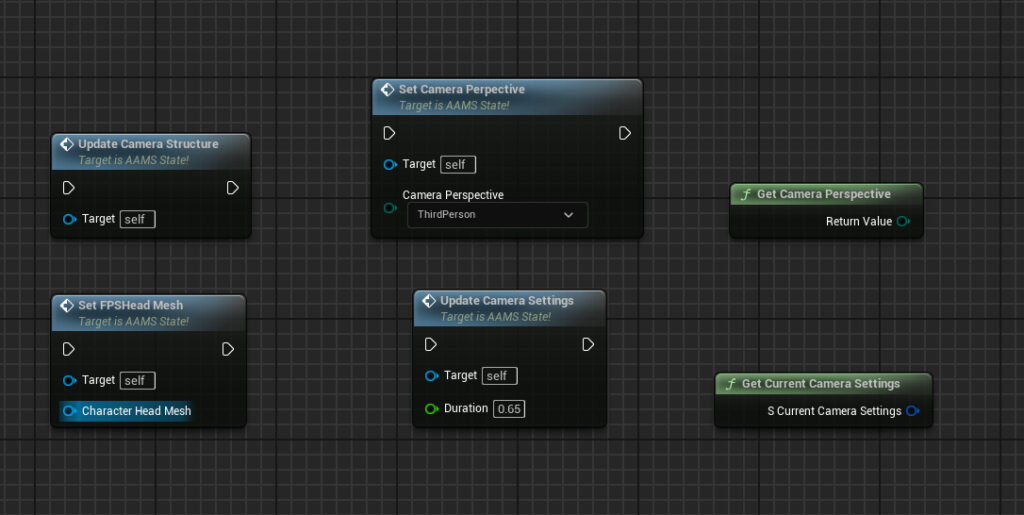
The camera settings in AAMS are configured in the State Component and override settings defined in the character blueprint. These settings can be updated per Movement State and based on Camera Perspective, using the Camera Settings Data Table. For more information, refer to the Camera Settings section of the documentation. There are four main camera functions in the State Component that you should be familiar with:
Set Camera Perspective #
The Set Camera Perspective function switches the camera between first-person and third-person perspectives. AAMS utilizes the base Unreal camera setup attached to a Spring Arm, so there’s no need for a second camera for FPS views.
Update Camera Structure #
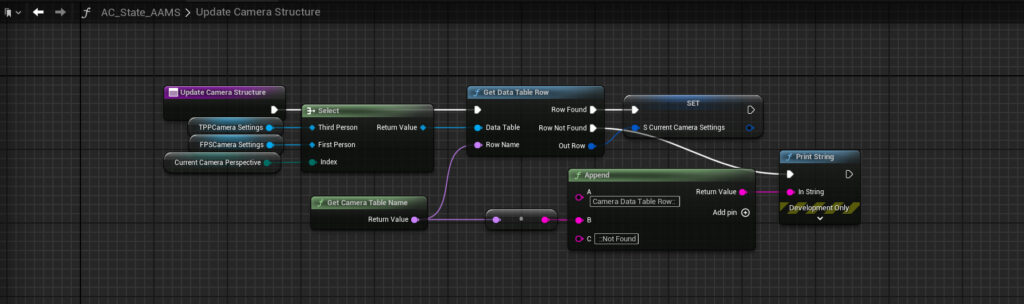
The Update Camera Structure function determines the current camera perspective and retrieves the appropriate Data Table variable. It then gets the current Movement State and sets the S_Current Camera Settings structure to the corresponding Camera Settings data table row. If the table row is not found, a print string will alert you to the missing row name.
Update Camera Settings #
The Update Camera Settings function is used in most movement events to update the camera. This function updates the camera structure to the appropriate slot, then adjusts the camera relative location, target arm length and offset, camera rotation, capsule radius, rotation clamp, camera lag, head mesh visibility, and control rotation yaw based on the settings in the accompanying camera settings data tables (see Camera Settings Section). The duration of the camera transition can also be set. The update uses a timeline, which can be lengthened or shortened to fit the desired transition length.
Set FPSHead Mesh #

The example character is divided into two meshes: body and head. The head mesh needs to be set for the State Component to control visibility when changing the camera perspective. This head mesh should be set either at BeginPlay or when the perspective is updated.
Default Camera Perspective and Data Tables #
To set the default camera perspective and the character’s appropriate camera settings data tables, select the State Component in the character blueprint, open the Details tab, and scroll to the Camera dropdown section. The default camera perspective can be set under “Perspective,” and the FPS and TPP data tables can be configured under “Settings.” Additionally, a simulated head bob can be enabled for the FPS perspective.
Passing Events #
Mechanics used in multiple Movement States are passed through the State Components to determine the current state and which actor component to communicate with, similar to the Determine Movement function. The main example of this is the Jump function in the State Component.
Jump #
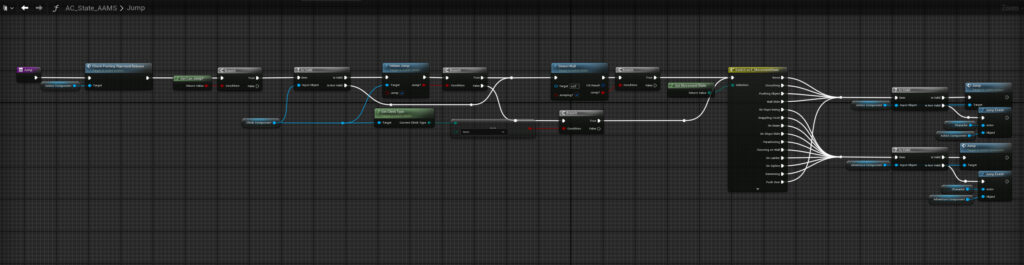
When Jump is pressed, a trace is run in front of the player to check for a climbable wall. If no wall is hit, a trace on the ground is activated to check for a ledge under the player. If either trace returns true, the Climb Component will initiate its Jump functions to check if the hit walls have climbable channels and are within the ranges to be climbed. If the Climb Component checks return false or the state wall traces return false, the State Component Jump function will determine the current Movement State and communicate with the appropriate Actor Component.
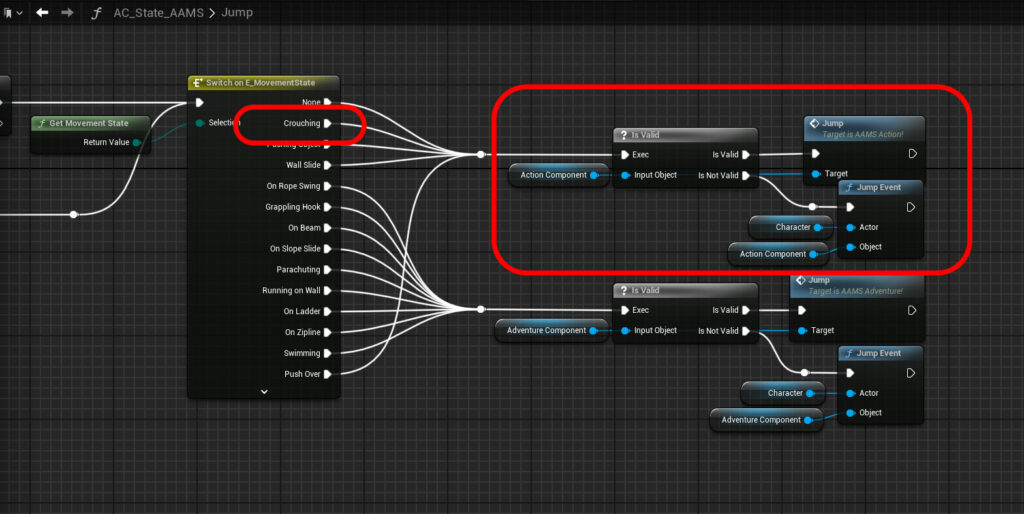
For example, if the player is crouching, the Jump function will check if the Action Component is valid in the player’s character blueprint, and if valid, it will communicate with the Action Component to initiate its jump function. To learn more about the Jump event in the Action Component, refer to the Jump/Double Jump subsection of the Action Component section of the documentation.
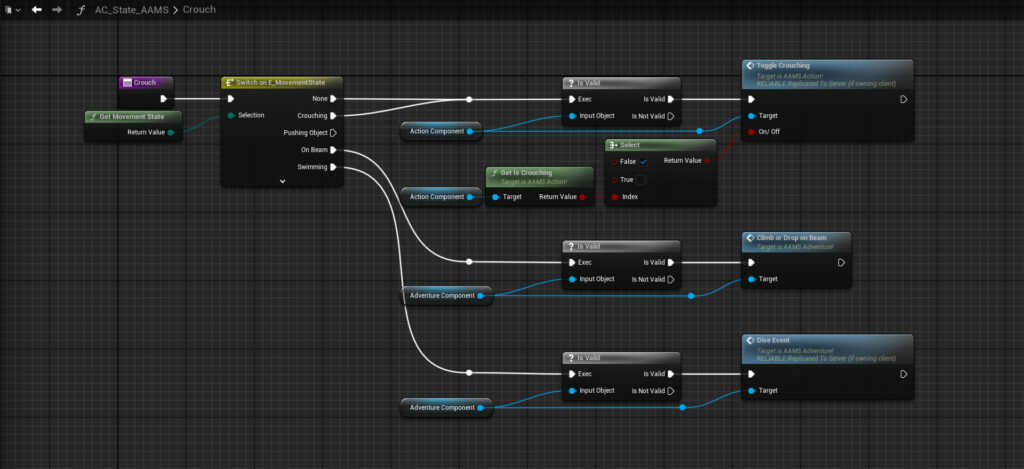
Crouch #
The crouch passing function is used to maintain a cohesive input key across Movement States and can be customized to fit your game keys. In the current setup, Crouch uses the same button as changing from hanging to walking on beams and diving under the surface while swimming. When pressing the Crouch key, the function checks the current Movement State and communicates with the appropriate actor component.
Perform Fatigue and Perform Death #
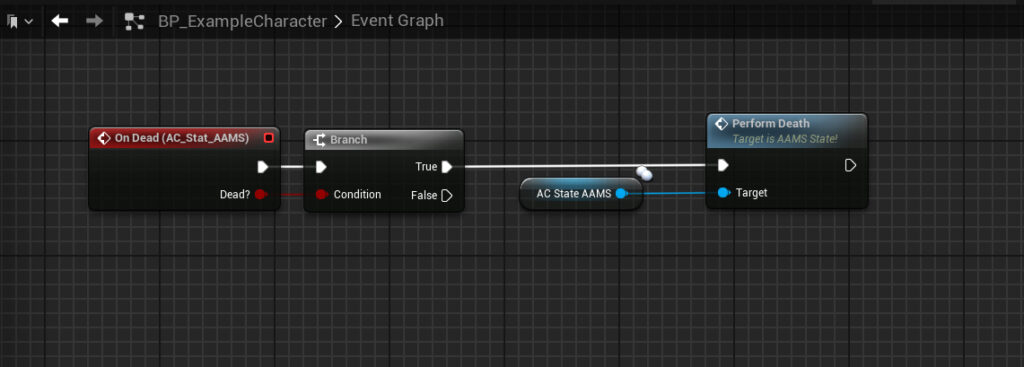
If you want to perform a special fatigue action when the player runs out of stamina or a special death action when out of health, you can create a function in the appropriate actor component and call it from the State Component. For example, a Swim Death event can be added to the Adventure Component, the same component housing the Swim mechanic. When the player runs out of the Breath stat while swimming, the Swim Death event is called in the Perform Death function. The Swim Death event triggers a drowning animation, then respawns the character. Examples of fatigue actions could include triggering a drop event when stamina is depleted during climbing.
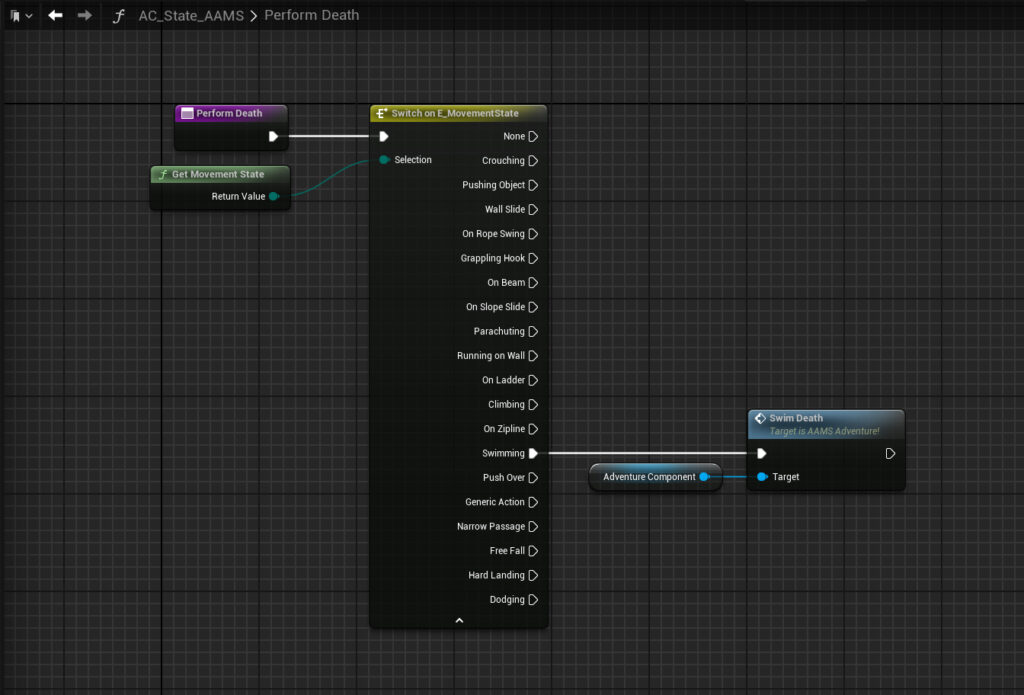
Toggle Sprint #
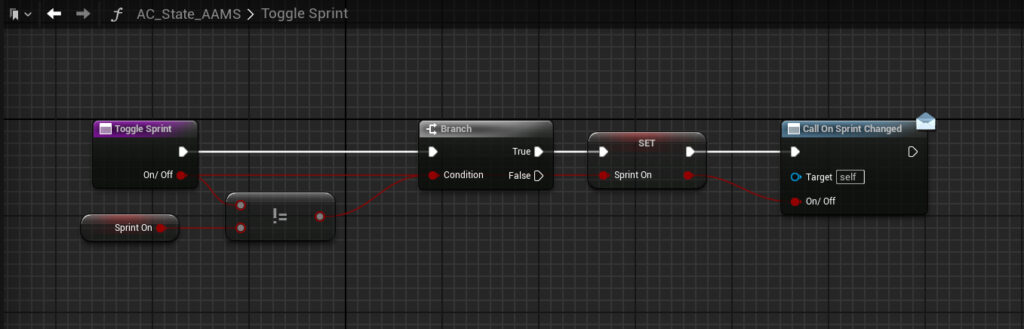
Sprint is passed through the State Component for the same reason as Crouching. The only difference is that Sprint triggers an Event Dispatcher bound to the appropriate blueprints where the sprint and sprint animations are set. For instance, in the Action Component, if the Current Movement State is set to None and the Current Move Speed is set to Walk or Jog, the player will start to sprint. If the Current Movement State is set to Swim, the player will start to swim fast.
Falling and Landing Checks #
Lastly, the State Component includes the Fall Timer and the On Landed event. The Fall Timer begins running as soon as the player jumps and monitors the character’s downward movement. When the character starts falling, the State Component alerts the other components that falling has begun, allowing for the appropriate traces to be initiated. The main checks include determining if free fall velocity has been reached and which free fall events to fire, checking if hard landing velocity has been reached, and assessing whether there are vine walls or ledges to grab onto.
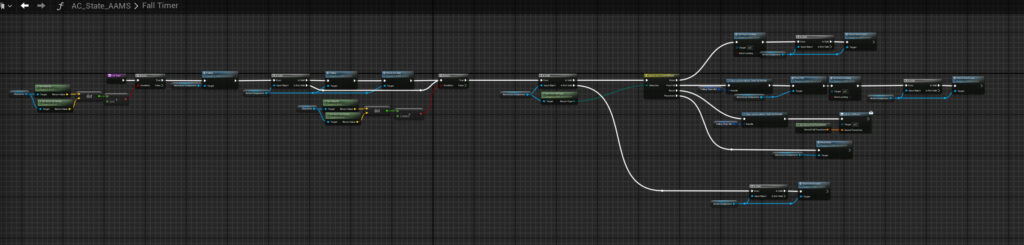
The On Landed event clears the falling timer and resets cameras, sound effects, and visual effects as needed.